SuperCom S7 Protocol Library
Software For The Digital Factory
High Performance Data Access to Siemens PLCs
Library, Component for S7 ISO-on-TCP Communication
S7 ISO-on-TCP Library
Windows
Library, Component
C#, C / C++, Delphi, Java, Pascal, Visual Basic, VB net
SuperCom Suite, SuperCom Serial Library incl. MODBUS, SuperCom for TCP/IP incl. MODBUS
ISO-on-TCP data communication to a S7 plc
Home
ADONTEC
Software Entwicklung, Serielle Daten Kommunikation, TCP/IP Client Server
Control S7 PLC with S7 protocol and ISO-on-TCP
SuperCom S7 Protocol Library
The SuperCom S7 Protocol Library includes functions to exchange data with a S7 PLC. The data transmission protocol used is referred as ISO-on-TCP (RFC 1006) and the connection to the PLC is established using the TCP/IP protocol.
The application is using the high-level functions of the SuperCom S7 Protocol Library for a flexible and speed optimized data exchange. Read or write operands like Data Blocks, Marker/Flags, Inputs, Outputs, Counters, Timer, Memory areas, etc.
Despite the professional orientation of the SuperCom S7 Protocol Library it is really easy to use and accompanied by many samples.
Exchanging data (Reading or Writing) are whenever possible handled internally as one request. This approach enables the SuperCom S7 Protocol Library to optimize speed and network traffic by treating requests for different plc areas as one so called "mixed requests" (one request send to the plc and one reply back).
Accomplish with ease
In most cases only a handful functions are needed to talk to the PLC. Your project is updated real fast. A lot of functions is backing you up to accomplish different tasks or configurations.
- Reliable data transfer
- Event driven: receive events and progress information while data exchanged
- Enables access to different operands within one request
- Real Fast Data Transfer
- Handle up to 255 connections simoultaneously
- Control up to 255 S7 PLC connections simoultaneously
- Access S7-200, S7-1200, S7-1500, S7-300, S7-400, S7 SoftSPS, WINAC RTX/WinLC and compatible
- "Mixed Requests" can additionally optimize speed and network traffic
- Low level Data monitoring and recording also supported.
The SuperCom S7 Protocol Library uses the SuperCom for TCP/IP communication layer which provides a rock solid foundation enabling to develop stable and accurate data communication software in short time. The special SuperCom data transfer technology used increases speed and data throughput and it often reduces network traffic.
The SuperCom S7 Protocol Library accesses the S7 station directly without using another software layer (e.g. OPC server or driver from third party) that can cause delays.
Samples:
1. Read input bits
C/C++
-- Init Sequence --
TCOMMID Com = RS_S7OpenLink(S7CONFIGSTR); // TCP setup and S7 setup
-- Access -- Fetch 3 input bits e.g. I1.0 to I1.2
BYTE cBuffer[3]; // 3 bits returned as 3 bytes
DWORD dwAmount = 3; // bits requested = 0, 1, 2
DWORD dwAddress = BitAddress(1, 0); // from Bit-Address
if (RS_S7Ready(Com))
{
long nRet = RS_S7Fetch(Com,
ORG_INPUT | ORG_REQ_IN_BITS,
dwAddress,
&dwAmount,
cBuffer);
}
:
RS_S7CloseLink(Com);
Delphi
-- Init Sequence --
Var Com:TCOMMID;
Com := RS_S7OpenLink(S7CONFIGSTR); // TCP setup and S7 setup
-- Access -- Fetch 3 input bits e.g. I1.0 to I1.2
Var cBuffer:Array[0..2] Of Byte; // 3 bits returned as 3 bytes
dwAmount:DWORD = 3; // bits requested = 0, 1, 2
dwAddress:DWORD = BitAddress(1, 0); // from Bit-Address
nRet:LongInt;
nRet := RS_S7Fetch(Com,
ORG_INPUT OR ORG_REQ_IN_BITS,
dwAddress,
dwAmount,
cBuffer);
:
RS_S7CloseLink(Com);
C#
-- Init Sequence --
Instead of the native API we demonstrate here the S7 class TSComS7
TSComS7 SCom = new TSComS7(S7CONFIGSTR);
SCom.S7Connect = true;
-- Access -- Fetch 3 input bits e.g. I1.0 to I1.2
if (SCom.S7Connected == false) return;
int Com = SCom.CommId; // parameter Com
byte[] cBuffer = new byte[3]; // 3 bits returned as 3 bytes
int dwAmount = 3; // bits requested = 0, 1, 2
int dwAddress = BitAddress(1, 0); // from Bit-Address
int nRet = SuperCom.S7ISOonTCP.RS_S7Fetch(Com,
ORG_INPUT | ORG_REQ_IN_BITS,
dwAddress,
ref dwAmount,
: cBuffer);
SCom.S7Connect = false;
Visual Basic
-- Init Sequence --
Var Com As Integer
Com = RS_S7OpenLink(S7CONFIGSTR) ' TCP setup and S7 setup
-- Access -- Fetch 3 input bits e.g. I1.0 to I1.2
Dim cBuffer As String ' 3 bits returned as 3 bytes
dwAmount = 3 ' bits requested = 0, 1, 2
dwAddress = BitAddress(1, 0) ' from Bit-Address
nRet = RS_S7Fetch(Com,
ORG_INPUT OR ORG_REQ_IN_BITS,
dwAddress,
dwAmount,
cBuffer)
:
RS_S7CloseLink(Com);
|
The above samples are nearly complete programs. More Init-Sequences can be found here.
RS_S7OpenLink is an extension to the newer function RS_OpenLink. The manual will also describe how to connect to the S7 using the native SuperCom API, but RS_S7OpenLink is definitely the shortest form.
2. Read from S7 Data Blocks
C/C++
// Fetch 2 WORDs from DB 10, starting at address 0
WORD wBuffer[2];
DWORD dwAddress = 0; // start from
DWORD dwAmount = 2 * sizeof(WORD); // 2*2 = 4 bytes
long nRet = RS_S7Fetch(Com,
RS_S7BLOCK_AREA_NR(ORG_DB, 10),
dwAddress,
&dwAmount,
wBuffer);
Delphi
// Fetch 2 WORDs from DB 10, starting at address 0
Var wBuffer:Array[0..1] Of WORD;
dwAddress:DWORD = 0; // start from
dwAmount:DWORD = 2 * SizeOf(WORD); // 2*2 = 4 bytes;
nRet := RS_S7Fetch(Com,
RS_S7BLOCK_AREA_NR(ORG_DB, 10),
dwAddress,
dwAmount,
wBuffer);
C#
// Fetch 2 WORDs from DB 10, starting at address 0
short[] wBuffer = new short[2];
int dwAddress = 0; // start from
int dwAmount = 2 * SizeOf(WORD); // 2*2 = 4 bytes;
int nRet = SuperCom.S7ISOonTCP.RS_S7Fetch(Com,
RS_S7BLOCK_AREA_NR(ORG_DB, 10),
dwAddress,
ref dwAmount,
wBuffer);
Visual Basic
// Fetch 2 WORDs from DB 10, starting at address 0
Dim wBuffer As String
dwAddress = 0 ' start from
dwAmount = 4 ' 2 WORD = 2*2=4 Bytes
nRet = RS_S7Fetch(Com,
RS_S7BLOCK_AREA_NR(ORG_DB, 10),
dwAddress,
dwAmount,
wBuffer)
|
License Information
Executable Applications (e.g. .EXE) developed using SuperCom can be distributed royalty free.
Supported compilers
C, C++, C#, Delphi, Java, Visual Studio, Visual C++, Visual Basic, Visual Basic NET (VB net), C++ Builder, Borland C/C++, Microsoft C/C++, MinGW, Borland Pascal, VBA, LabVIEW, PowerBuilder and other Windows programming tools (MS .NET ?).
Samples
for C, C++, C#, Delphi, Java, Visual Studio, Visual Basic 6, Visual Basic .NET (VB net) included. Many small samples also listed in the manual. More ...
How to use?
In order to use the SuperCom S7 Protocol Library a TCP/IP capable SuperCom library is also required e.g. combine with SuperCom for TCP/IP or SuperCom Suite. See also the following chart.
The SuperCom S7 Protocol Library can also be used to analyse and monitor error conditions on a PLC since polling of signals and values on short time frames is possible.
What to order?
Some possible combinations can be found here
PDF Document: SuperCom-S7-Software-Library 
S5 Protocol Suite
Protocols for S5 compatible data communication More...
|
Home Back
|
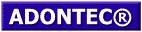 It Simply Works!
Updated on: 2024-05-16 15:29:14
|
|